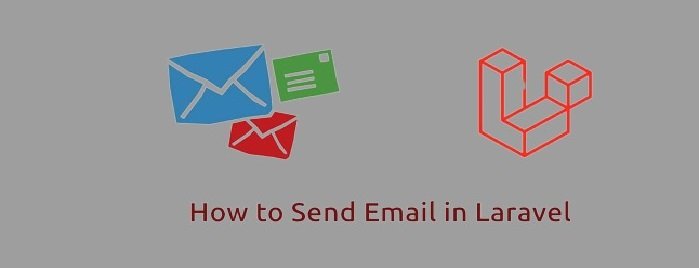
In this tutorial, we’ll walk through the process of sending a registration confirmation email in Laravel. Sending personalized confirmation emails enhances user experience and ensures successful user onboarding. We’ll cover the entire process from configuring the mail driver to customizing email templates.
Step 1: Configure Mail Driver
Before sending emails, make sure your mail driver is properly configured. Open the config/mail.php
file and update the necessary settings. You can use services like Mailgun, SMTP, etc. Configure the driver in your .env
file with the corresponding credentials.
Step 2: Create a Mailable
Generate a Mailable class using the Artisan command:
php artisan make:mail RegistrationMail
App\Mail
namespace.Step 3: Customize the Mailable
Open the generated RegistrationMail.php
file and customize the build
method to include the registration details. Define public properties for user details to be passed to the view.
Step 4: Create an Email Template
Create a Blade view file for the email content. Laravel expects this file to be in the resources/views/emails
directory. Create resources/views/emails/registration.blade.php
:
Step 5: Send Email in Registration Controller
In your user registration controller (e.g., RegisterController.php
), after creating a new user, dispatch the RegistrationMail
Mailable:
use App\Mail\RegistrationMail;
use Illuminate\Support\Facades\Mail;
Replace $user
with the actual variable containing the user details.
Congratulations! You’ve successfully implemented registration confirmation emails in your Laravel application. Users will now receive personalized emails with their registration details, enhancing their onboarding experience.